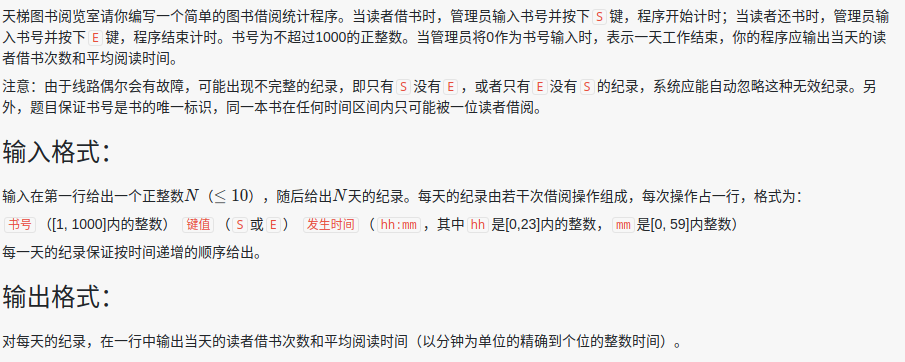
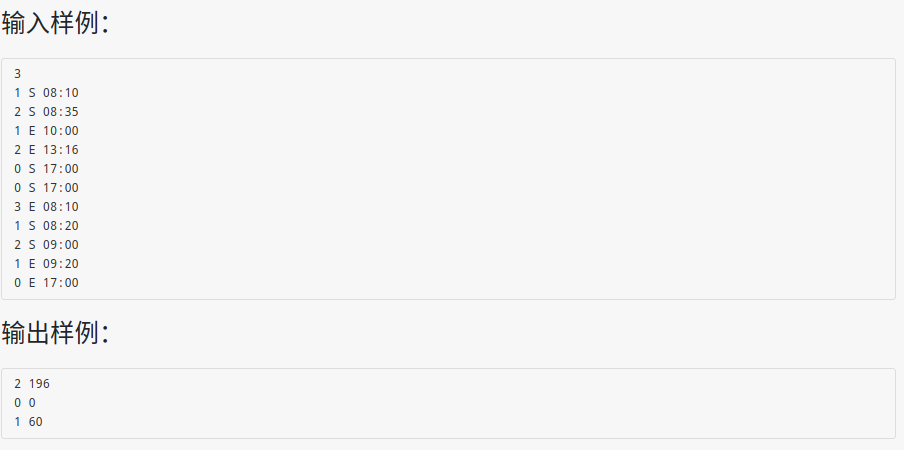
这道题要求一天内所有书的借阅次数和阅读时长,如果有些书只有借没有还,或者只有还没有借,则忽略不处理。
借书的时候书本不能被借阅,因此首先想到用结构体把书的属性写在一起。
- 巧妙之处是,通过结构体数组访问不同id的书的属性,且能通过标记思想限制一本书在一段时间内只能被一位读者借阅。
- 最后求平均阅读时间(阅读时间/阅读次数)可能会是浮点数,其中阅读时长和阅读次数都是整数,要*1.0转换成浮点数,并且+0.5进行四舍五入。根据题目要求,再进行强制类型转换成int。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| #include<iostream> #include <cstdio> using namespace std; typedef struct Book{ int start; int borrowed; }; Book book[1005]; int getTime(int h,int m) { int time = h*60+m; return time; } int main() { int n; cin >> n; int id,hours,mins,read_time,cnt; char op; for(int i=0;i<n;i++) { read_time = 0; cnt = 0; while(scanf("%d %c %d:%d",&id,&op,&hours,&mins)!=EOF) { if(id == 0) break; if(op == 'S') { book[id].start = getTime(hours,mins); book[id].borrowed = 1; } else if(op == 'E' && book[id].borrowed) { read_time += getTime(hours,mins) - book[id].start; cnt++; book[id].borrowed = 0; } } if(cnt) { cout << cnt << " " << int(read_time*1.0/cnt+0.5) <<endl; } else { cout << "0 0" <<endl; } } }
|
参考https://blog.csdn.net/qq_37729102/article/details/80721099